The XBeePacket class is the base class to implement XBee API frames (API Operations) More...
#include <xbeepacket.h>
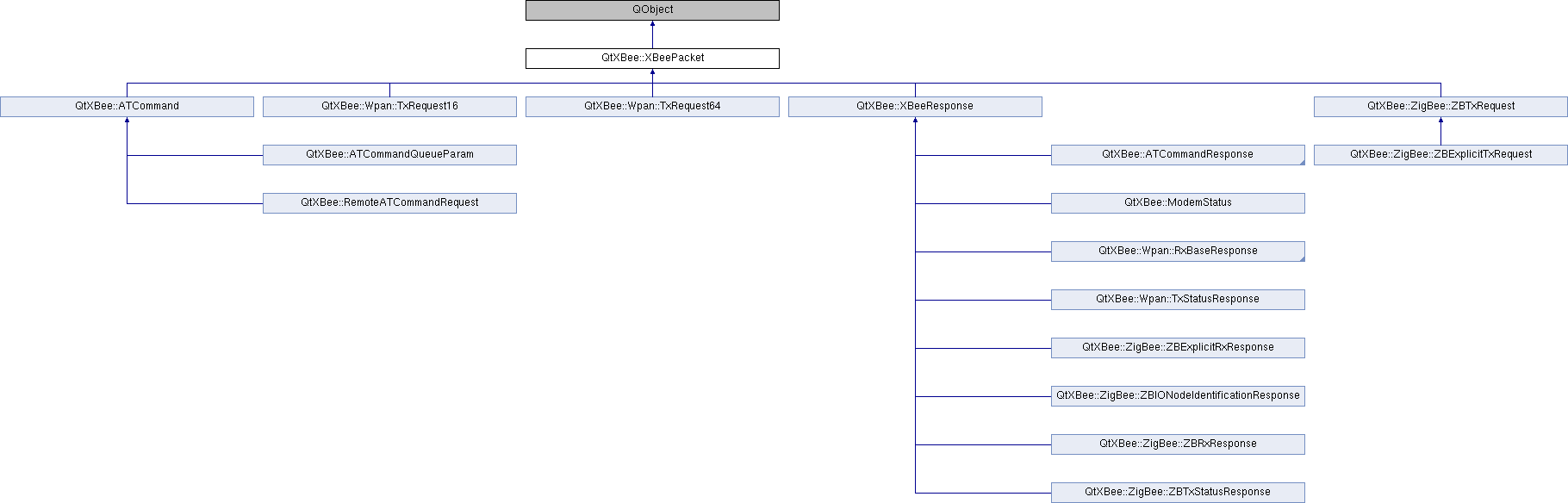
Public Types | |
enum | ApiId { TxRequest64Id = 0x00, TxRequest16Id = 0x01, ATCommandId = 0x08, ATCommandQueueId = 0x09, ZBTxRequestId = 0x10, ZBExplicitTxRequestId = 0x11, RemoteATCommandRequestId = 0x17, CreateSourceRouteId = 0x21, ZBRegisterJoiningDeviceId = 0x24, Rx64ResponseId = 0x80, Rx16ResponseId = 0x81, Rx64IOResponseId = 0x82, Rx16IOResponseId = 0x83, ATCommandResponseId = 0x88, TxStatusResponseId = 0x89, ModemStatusResponseId = 0x8A, ZBTxStatusResponseId = 0x8B, ZBRxResponseId = 0x90, ZBExplicitRxResponseId = 0x91, ZBIOSampleResponseId = 0x92, XBeeSensorReadIndicatorId = 0x94, ZBIONodeIdentificationId = 0x95, RemoteATCommandResponseId = 0x97, OverTheAirFirmwareUpdateId = 0xA0, RouteRecordIndicatorId = 0xA1, DeviceAuthenticatedIndicatorId = 0xA2, ManyToOneRouteRequestId = 0xA3, RegisterJoiningDeviceStatusId = 0xA4, JoinNotificationStatusId = 0xA5, UndefinedId = 0xFF } |
The ApiId enum identifies sent/received frame's type. More... | |
enum | SpecialByte { StartDelimiter = 0x7E, Escape = 0x7D, XON = 0x11, XOFF = 0x13 } |
The SpecialByte enum defines the special bytes. More... | |
Public Member Functions | |
XBeePacket (QObject *parent=0) | |
XBeePacket's constructor. More... | |
void | setStartDelimiter (unsigned sd) |
Sets the frame's start delimiter. By default the start delemiter is set to 0x7E. More... | |
void | setLength (unsigned length) |
Sets the frame's length. More... | |
void | setFrameType (ApiId type) |
Sets the frame's type. More... | |
void | setFrameId (quint8 id) |
Sets the frame's id. More... | |
void | setChecksum (unsigned cs) |
Sets the checksum. More... | |
bool | setPacket (const QByteArray &packet) |
Sets the packet's raw data. More... | |
QByteArray | packet () const |
Returns the frame's packet (raw data) More... | |
unsigned | startDelimiter () const |
Returns the frame's start delimiter. More... | |
quint16 | length () const |
Returns the frame-specific data length (Number of bytes between the length and the checksum) More... | |
ApiId | frameType () const |
Returns the frame's type. More... | |
quint8 | frameId () const |
Returns the frame's id. More... | |
unsigned | checksum () const |
Returns the packet's checksum. More... | |
virtual void | assemblePacket () |
Assembles the packet to be able to send it. More... | |
virtual void | clear () |
Clears the packet by reseting all attributes to their default value. More... | |
virtual QString | toString () |
Returns a debug string containing all packet's informations. More... | |
void | escapePacket () |
bool | unescapePacket () |
Static Public Member Functions | |
static QString | frameTypeToString (const ApiId type) |
Returns the given frame type ApiId into a human readable string. More... | |
Protected Member Functions | |
virtual bool | parseApiSpecificData (const QByteArray &data) |
Parses the packet API specific data. More... | |
void | createChecksum (QByteArray array) |
Computes the checksum of the given QByteArray, and set it. More... | |
Protected Attributes | |
QByteArray | m_packet |
Detailed Description
The XBeePacket class is the base class to implement XBee API frames (API Operations)
As an alternative to Transparent Operation, API (Application Programming Interface) Operations are available. API operation requires that communication with the module be done through a structured interface (data is communicated in frames in a defined order). The API specifies how commands, command responses and module status messages are sent and received from the module using a UART Data Frame.
- Todo:
- Create the XBeeRequest class, based on XBeePacket to implement the request packet specific part and remove them from XBeePacket (XBeePacket::assemblePacket(), ...)
Member Enumeration Documentation
The ApiId enum identifies sent/received frame's type.
Enumerator | |
---|---|
TxRequest64Id |
Identifies a Transmit request with a 64-bits addressing (Wpan::TxRequest64) |
TxRequest16Id |
Identifies a Transmit request with a 16-bits addressing (Wpan::TxRequest16) |
ATCommandId |
Identifies an AT Command Frame (ATCommand) |
ATCommandQueueId |
Identifies an AT Command - Queue Parameter Value Frame (ATCommandQueueParam) |
ZBTxRequestId |
Identifies a ZigBee Transmit Request Frame (ZigBee::ZBTxRequest) |
ZBExplicitTxRequestId |
Identifies an Explicit Addressing Command Frame (ZigBee::ZBExplicitTxRequest)
|
RemoteATCommandRequestId |
Identifies a Remote Command Request Frame (RemoteATCommandRequest) |
CreateSourceRouteId |
Identifies A Create Source Route Frame. This frame creates a source route in the module. A source route specifies the complete route a packet should traverse to get from source to destination. Source routing should be used with many-to-one routing for best results.
|
Rx64ResponseId |
Identifies a response (Wpan::TxRequest64) to a Wpan::TxRequest64 (XBeePacket::TxRequest64Id) |
Rx16ResponseId |
Identifies a response (Wpan::TxRequest16) to a Wpan::TxRequest16 (XBeePacket::TxRequest16Id) |
Rx64IOResponseId |
Identifies a Wpan::RxResponseIoSample64 |
Rx16IOResponseId |
Identifies a Wpan::RxResponseIoSample16 |
ATCommandResponseId |
Identifies an AT Command Response Frame (ATCommandResponse) |
TxStatusResponseId |
Identifies a Wpan::TxStatusResponse. When a Tx Request (Wpan::TxRequest64 or Wpan::TxRequest16) is completed, the module sends a Tx Status message. This message will indicate if the packet was transmitted successfully or if there was a failure. |
ModemStatusResponseId |
Identifies a Modem Status Frame (ModemStatus) |
ZBTxStatusResponseId |
Identifies a ZigBee Transmit Status (ZigBee::ZBTxStatusResponse) |
ZBRxResponseId |
Identifies a ZigBee Receive Packet Frame when AO=0 (ZigBee::ZBRxResponse) |
ZBExplicitRxResponseId |
Identifies a ZigBee Explicit Rx Indicator when AO=1 (ZigBee::ZBExplicitRxResponse) |
ZBIOSampleResponseId |
When the module receives an IO sample frame from a remote device, it sends the sample out the UART using this frame type (when AO=0). Only modules running API firmware will send IO samples out the UART. |
XBeeSensorReadIndicatorId |
When the module receives a sensor sample (from a Digi 1-wire sensor adapter), it is sent out the UART using this message type (when AO=0). |
ZBIONodeIdentificationId |
Identifies a Node Identification Indicator Frame (ZigBee::ZBIONodeIdentificationResponse) |
RemoteATCommandResponseId |
Identifies a Remote Command Response Frame (RemoteATCommandResponse) |
OverTheAirFirmwareUpdateId |
The Over-the-Air Firmware Update Status frame provides a status indication of a firmware update transmission attempt. If a query command (0x01 0x51) is sent to a target with a 64-bit address of 0x0013A200 40522BAA through an updater with 64-bit address 0x0013A200403E0750 and 16-bit address 0x0000, the following is the expected response. |
RouteRecordIndicatorId |
The route record indicator is received whenever a device sends a ZigBee route record command. This is used with many-to-one routing to create source routes for devices in a network. |
ManyToOneRouteRequestId |
The many-to-one route request indicator frame is sent out the UART whenever a many-to-one route request is received. |
UndefinedId |
Undefined frame. Invalid value. |
Constructor & Destructor Documentation
|
explicit |
XBeePacket's constructor.
- Parameters
-
parent
Member Function Documentation
|
virtual |
Assembles the packet to be able to send it.
This function is called by the XBee class, when the packet in being sent.
- Note
- Overload this function to create your own packet.
Reimplemented in QtXBee::ATCommand, QtXBee::RemoteATCommandRequest, QtXBee::ZigBee::ZBTxRequest, QtXBee::Wpan::TxRequest16, and QtXBee::Wpan::TxRequest64.
unsigned QtXBee::XBeePacket::checksum | ( | ) | const |
|
virtual |
Clears the packet by reseting all attributes to their default value.
- Note
- All subclass should reimplement this method.
Reimplemented in QtXBee::ATCommand, QtXBee::Wpan::RxResponseIoSampleBase, QtXBee::ATCommandResponse, QtXBee::RemoteATCommandRequest, QtXBee::Wpan::TxStatusResponse, QtXBee::RemoteATCommandResponse, QtXBee::Wpan::RxResponse16, QtXBee::Wpan::RxResponse64, QtXBee::Wpan::TxRequest16, QtXBee::Wpan::TxRequest64, QtXBee::Wpan::RxBaseResponse, and QtXBee::XBeeResponse.
|
protected |
Computes the checksum of the given QByteArray, and set it.
- Parameters
-
array
quint8 QtXBee::XBeePacket::frameId | ( | ) | const |
XBeePacket::ApiId QtXBee::XBeePacket::frameType | ( | ) | const |
Returns the frame's type.
- Returns
- the frame's type
- See Also
- XBeePacket::setFrameType()
- XBeePacket::APIFrameType
|
static |
Returns the given frame type ApiId into a human readable string.
- Returns
- the given frame type ApiId into a human readable string
- Parameters
-
type
- See Also
- ApiId
quint16 QtXBee::XBeePacket::length | ( | ) | const |
Returns the frame-specific data length (Number of bytes between the length and the checksum)
- Returns
- the packet's length
- See Also
- XBeePacket::setLength()
QByteArray QtXBee::XBeePacket::packet | ( | ) | const |
Returns the frame's packet (raw data)
- Returns
- the frame's packet (raw data)
|
protectedvirtual |
Parses the packet API specific data.
Are conciderated as packet specific data, the data between API frame Id and checksum.
For example, the AT command response frame:
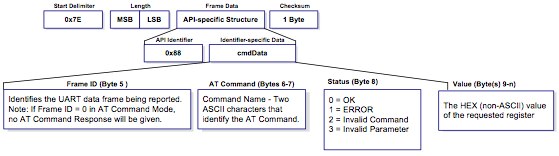
The specific part is bytes [6 - (length() -1)] (AT command, Status, Value)
- Parameters
-
data
- Returns
- true if succeeded; false otherwise.
- Note
- All subclasses must reimplement this method, which is called by XBeePacket::setPacket()
Reimplemented in QtXBee::Wpan::RxResponseIoSampleBase, QtXBee::ATCommandResponse, QtXBee::Wpan::TxStatusResponse, QtXBee::RemoteATCommandResponse, QtXBee::Wpan::RxResponse16, and QtXBee::Wpan::RxResponse64.
void QtXBee::XBeePacket::setChecksum | ( | unsigned | cs | ) |
Sets the checksum.
- Parameters
-
cs
- See Also
- XBeePacket::checksum()
- Todo:
- Does it make sense that this method is public ? If not, make it protected.
void QtXBee::XBeePacket::setFrameId | ( | quint8 | id | ) |
void QtXBee::XBeePacket::setFrameType | ( | ApiId | type | ) |
Sets the frame's type.
- Parameters
-
type
- See Also
- XBeePacket::frameType()
- Todo:
- Does it make sense that this method is public ? If not, make it protected.
void QtXBee::XBeePacket::setLength | ( | unsigned | length | ) |
Sets the frame's length.
- Parameters
-
length
- See Also
- XBeePacket::length()
- Todo:
- Does it make sense that this method is public ? If not, make it protected.
bool QtXBee::XBeePacket::setPacket | ( | const QByteArray & | packet | ) |
Sets the packet's raw data.
- Parameters
-
packet
- Returns
- true if succeeded; false otherwise.
void QtXBee::XBeePacket::setStartDelimiter | ( | unsigned | sd | ) |
Sets the frame's start delimiter. By default the start delemiter is set to 0x7E.
- See Also
- XBeePacket::startDelimiter()
- Parameters
-
sd
unsigned QtXBee::XBeePacket::startDelimiter | ( | ) | const |
Returns the frame's start delimiter.
- Returns
- the frame's start delimiter
- See Also
- XBeePacket::setStartDelimiter()
- Todo:
- Does it make sense that this method is public ? If not, make it protected.
|
virtual |
Returns a debug string containing all packet's informations.
- Returns
- a debug string containing all packet's informations.
Reimplemented in QtXBee::ATCommand, QtXBee::Wpan::RxResponseIoSampleBase, QtXBee::ATCommandResponse, QtXBee::RemoteATCommandRequest, QtXBee::Wpan::TxStatusResponse, QtXBee::ModemStatus, QtXBee::RemoteATCommandResponse, QtXBee::Wpan::RxResponse16, QtXBee::Wpan::RxResponse64, QtXBee::Wpan::TxRequest16, QtXBee::Wpan::TxRequest64, QtXBee::Wpan::RxBaseResponse, and QtXBee::ZigBee::ZBIONodeIdentificationResponse.
Member Data Documentation
|
protected |
Contains the packet's data (sent or received)
The documentation for this class was generated from the following files:
- /home/thomas/Projects/QtXBee/src/qtxb/xbeepacket.h
- /home/thomas/Projects/QtXBee/src/qtxb/xbeepacket.cpp
- pages/xbeepacket.dox