In response to an ATCommand message, the module will send an ATCommandResponse message. More...
#include <atcommandresponse.h>
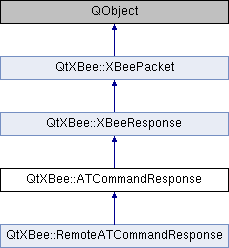
Public Types | |
enum | Status { Ok = 0, Error = 1, InvalidCommand = 2, InvalidParameter = 3, TxFailure = 4 } |
The CommandStatus enum. More... | |
Public Member Functions | |
ATCommandResponse (QObject *parent=0) | |
ATCommandResponse (const QByteArray &packet, QObject *parent=0) | |
virtual QString | toString () |
Returns a debug string containing all packet's informations. More... | |
virtual void | clear () |
Clears the packet by reseting all attributes to their default value. More... | |
void | setATCommand (ATCommand::ATCommandType at) |
Sets the response's AT Command. More... | |
void | setATCommand (const QByteArray &at) |
Sets the response's AT Command. More... | |
void | setStatus (const Status status) |
Sets the command status. More... | |
ATCommand::ATCommandType | atCommand () const |
Returns the response's AT Command. More... | |
Status | status () const |
Returns the command' status. More... | |
QString | statusToString () const |
Returns the ATCommandResponse::Status as a human readable string. More... | |
![]() | |
XBeeResponse (QObject *parent=0) | |
XBeeResponse's constructor. More... | |
~XBeeResponse () | |
XBeeResponse's destructor. | |
void | setData (const QByteArray &data) |
Sets the response's data. More... | |
QByteArray | data () const |
Returns the response's data. More... | |
![]() | |
XBeePacket (QObject *parent=0) | |
XBeePacket's constructor. More... | |
void | setStartDelimiter (unsigned sd) |
Sets the frame's start delimiter. By default the start delemiter is set to 0x7E. More... | |
void | setLength (unsigned length) |
Sets the frame's length. More... | |
void | setFrameType (ApiId type) |
Sets the frame's type. More... | |
void | setFrameId (quint8 id) |
Sets the frame's id. More... | |
void | setChecksum (unsigned cs) |
Sets the checksum. More... | |
bool | setPacket (const QByteArray &packet) |
Sets the packet's raw data. More... | |
QByteArray | packet () const |
Returns the frame's packet (raw data) More... | |
unsigned | startDelimiter () const |
Returns the frame's start delimiter. More... | |
quint16 | length () const |
Returns the frame-specific data length (Number of bytes between the length and the checksum) More... | |
ApiId | frameType () const |
Returns the frame's type. More... | |
quint8 | frameId () const |
Returns the frame's id. More... | |
unsigned | checksum () const |
Returns the packet's checksum. More... | |
virtual void | assemblePacket () |
Assembles the packet to be able to send it. More... | |
void | escapePacket () |
bool | unescapePacket () |
Static Public Member Functions | |
static QString | statusToString (const ATCommandResponse::Status status) |
Returns the given ATCommandResponse::Status as a human readable string. More... | |
Protected Member Functions | |
virtual bool | parseApiSpecificData (const QByteArray &data) |
Parses the packet API specific data. More... | |
Protected Attributes | |
ATCommand::ATCommandType | m_atCommand |
Status | m_status |
![]() | |
QByteArray | m_data |
![]() | |
QByteArray | m_packet |
Detailed Description
In response to an ATCommand message, the module will send an ATCommandResponse message.
Some commands will send back multiple frames (for example, the ND (Node Discover) command).
API identifier value: 0x88
- See Also
- ATCommand
Member Enumeration Documentation
Member Function Documentation
ATCommand::ATCommandType QtXBee::ATCommandResponse::atCommand | ( | ) | const |
Returns the response's AT Command.
- Returns
- the response's AT Command
|
virtual |
Clears the packet by reseting all attributes to their default value.
- Note
- All subclass should reimplement this method.
Reimplemented from QtXBee::XBeeResponse.
Reimplemented in QtXBee::RemoteATCommandResponse.
|
protectedvirtual |
Parses the packet API specific data.
Are conciderated as packet specific data, the data between API frame Id and checksum.
For example, the AT command response frame:
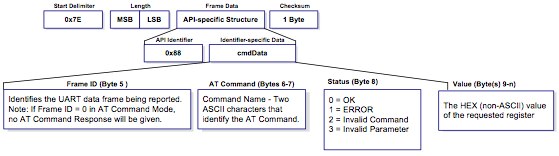
The specific part is bytes [6 - (length() -1)] (AT command, Status, Value)
- Parameters
-
data
- Returns
- true if succeeded; false otherwise.
- Note
- All subclasses must reimplement this method, which is called by XBeePacket::setPacket()
Reimplemented from QtXBee::XBeePacket.
Reimplemented in QtXBee::RemoteATCommandResponse.
void QtXBee::ATCommandResponse::setATCommand | ( | ATCommand::ATCommandType | at | ) |
void QtXBee::ATCommandResponse::setATCommand | ( | const QByteArray & | at | ) |
Sets the response's AT Command.
- Parameters
-
at
void QtXBee::ATCommandResponse::setStatus | ( | const Status | status | ) |
Sets the command status.
- Parameters
-
status
- See Also
- ATCommandResponse::CommandStatus
ATCommandResponse::Status QtXBee::ATCommandResponse::status | ( | ) | const |
Returns the command' status.
- Returns
- the command' status
- See Also
- ATCommandResponse::CommandStatus
QString QtXBee::ATCommandResponse::statusToString | ( | ) | const |
Returns the ATCommandResponse::Status as a human readable string.
- Returns
- the given ATCommandResponse::Status as a human readable string
- See Also
- ATCommandResponse::Status
|
static |
Returns the given ATCommandResponse::Status as a human readable string.
- Parameters
-
status
- Returns
- the given ATCommandResponse::Status as a human readable string
- See Also
- ATCommandResponse::Status
|
virtual |
Returns a debug string containing all packet's informations.
- Returns
- a debug string containing all packet's informations.
Reimplemented from QtXBee::XBeePacket.
Reimplemented in QtXBee::RemoteATCommandResponse.
The documentation for this class was generated from the following files:
- /home/thomas/Projects/QtXBee/src/qtxb/atcommandresponse.h
- /home/thomas/Projects/QtXBee/src/qtxb/atcommandresponse.cpp