The TxStatusResponse class is used to indicate if a packet (TxRequest64 or TxRequest16) was transmitted successfully or if there was a failure. More...
#include <txstatusresponse.h>
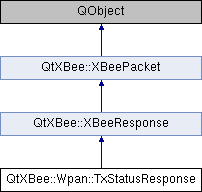
Public Types | |
enum | Status { Unknown = -1, Success = 0, NoACK = 1, CCAFailure = 2, Purged = 3 } |
The Status enum. More... | |
Public Member Functions | |
TxStatusResponse (QObject *parent=0) | |
virtual void | clear () |
Clears the packet by reseting all attributes to their default value. More... | |
virtual QString | toString () |
Returns a debug string containing all packet's informations. More... | |
void | setStatus (const Status status) |
Sets the transmit status. More... | |
Status | status () const |
Returns the transmit status. More... | |
![]() | |
XBeeResponse (QObject *parent=0) | |
XBeeResponse's constructor. More... | |
~XBeeResponse () | |
XBeeResponse's destructor. | |
void | setData (const QByteArray &data) |
Sets the response's data. More... | |
QByteArray | data () const |
Returns the response's data. More... | |
![]() | |
XBeePacket (QObject *parent=0) | |
XBeePacket's constructor. More... | |
void | setStartDelimiter (unsigned sd) |
Sets the frame's start delimiter. By default the start delemiter is set to 0x7E. More... | |
void | setLength (unsigned length) |
Sets the frame's length. More... | |
void | setFrameType (ApiId type) |
Sets the frame's type. More... | |
void | setFrameId (quint8 id) |
Sets the frame's id. More... | |
void | setChecksum (unsigned cs) |
Sets the checksum. More... | |
bool | setPacket (const QByteArray &packet) |
Sets the packet's raw data. More... | |
QByteArray | packet () const |
Returns the frame's packet (raw data) More... | |
unsigned | startDelimiter () const |
Returns the frame's start delimiter. More... | |
quint16 | length () const |
Returns the frame-specific data length (Number of bytes between the length and the checksum) More... | |
ApiId | frameType () const |
Returns the frame's type. More... | |
quint8 | frameId () const |
Returns the frame's id. More... | |
unsigned | checksum () const |
Returns the packet's checksum. More... | |
virtual void | assemblePacket () |
Assembles the packet to be able to send it. More... | |
void | escapePacket () |
bool | unescapePacket () |
Static Public Member Functions | |
static QString | statusToString (const Status status) |
Returns the Status as a human readable string. More... | |
Protected Member Functions | |
virtual bool | parseApiSpecificData (const QByteArray &data) |
Parses the packet API specific data. More... | |
Additional Inherited Members | |
![]() | |
QByteArray | m_data |
Detailed Description
The TxStatusResponse class is used to indicate if a packet (TxRequest64 or TxRequest16) was transmitted successfully or if there was a failure.
API identifier value: 0x89
Member Enumeration Documentation
Member Function Documentation
|
virtual |
Clears the packet by reseting all attributes to their default value.
- Note
- All subclass should reimplement this method.
Reimplemented from QtXBee::XBeeResponse.
|
protectedvirtual |
Parses the packet API specific data.
Are conciderated as packet specific data, the data between API frame Id and checksum.
For example, the AT command response frame:
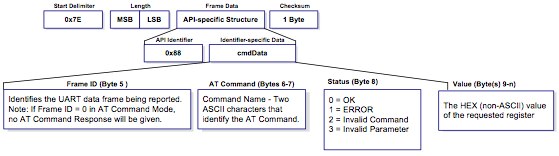
The specific part is bytes [6 - (length() -1)] (AT command, Status, Value)
- Parameters
-
data
- Returns
- true if succeeded; false otherwise.
- Note
- All subclasses must reimplement this method, which is called by XBeePacket::setPacket()
Reimplemented from QtXBee::XBeePacket.
void QtXBee::Wpan::TxStatusResponse::setStatus | ( | const Status | status | ) |
Sets the transmit status.
- Parameters
-
status
TxStatusResponse::Status QtXBee::Wpan::TxStatusResponse::status | ( | ) | const |
Returns the transmit status.
- Returns
- the transmit status
|
static |
Returns the Status as a human readable string.
- Parameters
-
status
- Returns
- the Status as a human readable string
|
virtual |
Returns a debug string containing all packet's informations.
- Returns
- a debug string containing all packet's informations.
Reimplemented from QtXBee::XBeePacket.
The documentation for this class was generated from the following files:
- /home/thomas/Projects/QtXBee/src/qtxb/wpan/txstatusresponse.h
- /home/thomas/Projects/QtXBee/src/qtxb/wpan/txstatusresponse.cpp