The TxRequest64 class is used to send a message over 802.15.4 network using 64 bits addresses. More...
#include <txrequest64.h>
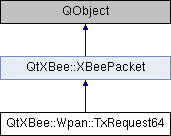
Public Member Functions | |
TxRequest64 (QObject *parent=0) | |
virtual void | assemblePacket () |
Assembles the packet to be able to send it. More... | |
virtual void | clear () |
Clears the packet by reseting all attributes to their default value. More... | |
virtual QString | toString () |
Returns a debug string containing all packet's informations. More... | |
void | setDestinationAddress (const quint64 address) |
Sets the 64 bits packet's destination address. More... | |
void | setData (const QByteArray &data) |
Sets the data to be sent (Up to 100 bytes per packet) More... | |
quint64 | destinationAddress () const |
Returns the packet's destination address. More... | |
QByteArray | data () const |
Returns the data to be sent. More... | |
![]() | |
XBeePacket (QObject *parent=0) | |
XBeePacket's constructor. More... | |
void | setStartDelimiter (unsigned sd) |
Sets the frame's start delimiter. By default the start delemiter is set to 0x7E. More... | |
void | setLength (unsigned length) |
Sets the frame's length. More... | |
void | setFrameType (ApiId type) |
Sets the frame's type. More... | |
void | setFrameId (quint8 id) |
Sets the frame's id. More... | |
void | setChecksum (unsigned cs) |
Sets the checksum. More... | |
bool | setPacket (const QByteArray &packet) |
Sets the packet's raw data. More... | |
QByteArray | packet () const |
Returns the frame's packet (raw data) More... | |
unsigned | startDelimiter () const |
Returns the frame's start delimiter. More... | |
quint16 | length () const |
Returns the frame-specific data length (Number of bytes between the length and the checksum) More... | |
ApiId | frameType () const |
Returns the frame's type. More... | |
quint8 | frameId () const |
Returns the frame's id. More... | |
unsigned | checksum () const |
Returns the packet's checksum. More... | |
void | escapePacket () |
bool | unescapePacket () |
Additional Inherited Members | |
![]() | |
enum | ApiId { TxRequest64Id = 0x00, TxRequest16Id = 0x01, ATCommandId = 0x08, ATCommandQueueId = 0x09, ZBTxRequestId = 0x10, ZBExplicitTxRequestId = 0x11, RemoteATCommandRequestId = 0x17, CreateSourceRouteId = 0x21, ZBRegisterJoiningDeviceId = 0x24, Rx64ResponseId = 0x80, Rx16ResponseId = 0x81, Rx64IOResponseId = 0x82, Rx16IOResponseId = 0x83, ATCommandResponseId = 0x88, TxStatusResponseId = 0x89, ModemStatusResponseId = 0x8A, ZBTxStatusResponseId = 0x8B, ZBRxResponseId = 0x90, ZBExplicitRxResponseId = 0x91, ZBIOSampleResponseId = 0x92, XBeeSensorReadIndicatorId = 0x94, ZBIONodeIdentificationId = 0x95, RemoteATCommandResponseId = 0x97, OverTheAirFirmwareUpdateId = 0xA0, RouteRecordIndicatorId = 0xA1, DeviceAuthenticatedIndicatorId = 0xA2, ManyToOneRouteRequestId = 0xA3, RegisterJoiningDeviceStatusId = 0xA4, JoinNotificationStatusId = 0xA5, UndefinedId = 0xFF } |
The ApiId enum identifies sent/received frame's type. More... | |
enum | SpecialByte { StartDelimiter = 0x7E, Escape = 0x7D, XON = 0x11, XOFF = 0x13 } |
The SpecialByte enum defines the special bytes. More... | |
![]() | |
static QString | frameTypeToString (const ApiId type) |
Returns the given frame type ApiId into a human readable string. More... | |
![]() | |
virtual bool | parseApiSpecificData (const QByteArray &data) |
Parses the packet API specific data. More... | |
void | createChecksum (QByteArray array) |
Computes the checksum of the given QByteArray, and set it. More... | |
![]() | |
QByteArray | m_packet |
Detailed Description
The TxRequest64 class is used to send a message over 802.15.4 network using 64 bits addresses.
API identifier value: 0x00
Member Function Documentation
|
virtual |
Assembles the packet to be able to send it.
This function is called by the XBee class, when the packet in being sent.
- Note
- Overload this function to create your own packet.
- Todo:
- Handle Options
- Todo:
- Check data's size (up to 100 bytes per packet)
Reimplemented from QtXBee::XBeePacket.
|
virtual |
Clears the packet by reseting all attributes to their default value.
- Note
- All subclass should reimplement this method.
Reimplemented from QtXBee::XBeePacket.
QByteArray QtXBee::Wpan::TxRequest64::data | ( | ) | const |
Returns the data to be sent.
- Returns
- the data to be sent
quint64 QtXBee::Wpan::TxRequest64::destinationAddress | ( | ) | const |
Returns the packet's destination address.
- Returns
- the packet's destination address.
void QtXBee::Wpan::TxRequest64::setData | ( | const QByteArray & | data | ) |
Sets the data to be sent (Up to 100 bytes per packet)
- Parameters
-
data the data to be sent
void QtXBee::Wpan::TxRequest64::setDestinationAddress | ( | const quint64 | address | ) |
Sets the 64 bits packet's destination address.
- Note
- To send the packet on the broadcast set the address to 0x000000000000FFFF
- Parameters
-
address the destination address
|
virtual |
Returns a debug string containing all packet's informations.
- Returns
- a debug string containing all packet's informations.
Reimplemented from QtXBee::XBeePacket.
The documentation for this class was generated from the following files:
- /home/thomas/Projects/QtXBee/src/qtxb/wpan/txrequest64.h
- /home/thomas/Projects/QtXBee/src/qtxb/wpan/txrequest64.cpp