The RemoteATCommandRequest class is used to query or set module parameters on a remote device. More...
#include <remoteatcommandrequest.h>
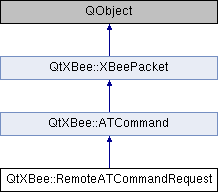
Public Types | |
enum | RemoteCommandOption { DisableRetriesAndRouteRepair = 0x01, ApplyChanges = 0x02, EnabledAPSEncryption = 0x20, UseExtendedTxTimeout = 0x40 } |
The RemoteCommandOption enum defines the remote command options. More... | |
![]() | |
enum | ATCommandType { ATUndefined = 0, ATWR = 0x5752, ATRE = 0x5245, ATFR = 0x4652, ATDH = 0x4448, ATDL = 0x444C, ATMY = 0x4D59, ATMP = 0x4D50, ATNC = 0x4E43, ATSH = 0x5348, ATSL = 0x534C, ATNI = 0x4E49, ATSE = 0x5345, ATDE = 0x4445, ATCI = 0x4349, ATTO = 0x544F, ATNP = 0x4E50, ATDD = 0x4444, ATCR = 0x4352, ATCH = 0x4348, ATDA = 0x4441, ATID = 0x4944, ATFP = 0x4650, ATAS = 0x4153, ATED = 0x4544, ATOP = 0x4F50, ATRR = 0x5252, ATRN = 0x524E, ATMM = 0x4D4D, ATCE = 0x4345, ATNH = 0x4E48, ATBH = 0x4248, ATOI = 0x4F49, ATNT = 0x4E54, ATNO = 0x4E4F, ATSC = 0x5343, ATSD = 0x5344, ATZS = 0x5A53, ATNJ = 0x4E4A, ATJV = 0x4A56, ATNW = 0x4E57, ATJN = 0x4A4E, ATAR = 0x4152, ATA1 = 0x4131, ATA2 = 0x4132, ATEE = 0x4545, ATEO = 0x454F, ATNK = 0x4E4B, ATKY = 0x4B59, ATPL = 0x504C, ATCA = 0x4341, ATPM = 0x504D, ATDB = 0x4442, ATPP = 0x5050, ATAP = 0x4150, ATAO = 0x414F, ATBD = 0x4244, ATNB = 0x4E42, ATSB = 0x5342, ATRO = 0x524F, ATIR = 0x4952, ATIC = 0x4943, ATP0 = 0x5030, ATP1 = 0x5031, ATP2 = 0x5032, ATP3 = 0x5033, ATD0 = 0x4430, ATD1 = 0x4431, ATD2 = 0x4432, ATD3 = 0x4433, ATD4 = 0x4434, ATD5 = 0x4435, ATD6 = 0x4436, ATD7 = 0x4437, ATD8 = 0x4438, ATLT = 0x4C54, ATPR = 0x5052, ATRP = 0x5250, ATV = 0x2556, ATVP = 0x562B, ATTP = 0x5450, ATVR = 0x5652, ATHV = 0x4856, ATAI = 0x4149, ATCT = 0x4354, ATCN = 0x434E, ATGT = 0x4754, ATCC = 0x4343, ATSM = 0x534D, ATSN = 0x534E, ATSP = 0x5350, ATDP = 0x4450, ATST = 0x5354, ATSO = 0x534F, ATWH = 0x5748, ATSI = 0x5349, ATPO = 0x504F, ATAC = 0x4143, ATNR = 0x4E52, ATCB = 0x4342, ATND = 0x4E44, ATDN = 0x444E, ATIS = 0x4953, AT1S = 0x3153 } |
The ATCommand enum defines the AT commands codes. More... | |
![]() | |
enum | ApiId { TxRequest64Id = 0x00, TxRequest16Id = 0x01, ATCommandId = 0x08, ATCommandQueueId = 0x09, ZBTxRequestId = 0x10, ZBExplicitTxRequestId = 0x11, RemoteATCommandRequestId = 0x17, CreateSourceRouteId = 0x21, ZBRegisterJoiningDeviceId = 0x24, Rx64ResponseId = 0x80, Rx16ResponseId = 0x81, Rx64IOResponseId = 0x82, Rx16IOResponseId = 0x83, ATCommandResponseId = 0x88, TxStatusResponseId = 0x89, ModemStatusResponseId = 0x8A, ZBTxStatusResponseId = 0x8B, ZBRxResponseId = 0x90, ZBExplicitRxResponseId = 0x91, ZBIOSampleResponseId = 0x92, XBeeSensorReadIndicatorId = 0x94, ZBIONodeIdentificationId = 0x95, RemoteATCommandResponseId = 0x97, OverTheAirFirmwareUpdateId = 0xA0, RouteRecordIndicatorId = 0xA1, DeviceAuthenticatedIndicatorId = 0xA2, ManyToOneRouteRequestId = 0xA3, RegisterJoiningDeviceStatusId = 0xA4, JoinNotificationStatusId = 0xA5, UndefinedId = 0xFF } |
The ApiId enum identifies sent/received frame's type. More... | |
enum | SpecialByte { StartDelimiter = 0x7E, Escape = 0x7D, XON = 0x11, XOFF = 0x13 } |
The SpecialByte enum defines the special bytes. More... | |
Public Member Functions | |
RemoteATCommandRequest (QObject *parent=0) | |
RemoteATCommandRequest's constructor. More... | |
virtual void | assemblePacket () |
Assembles the packet to be able to send it. More... | |
virtual void | clear () |
Clears the packet by reseting all attributes to their default value. More... | |
virtual QString | toString () |
Returns a debug string containing all packet's informations. More... | |
void | setDestinationAddress64 (const quint64 dest) |
Sets the 64-bits address of the destination device. More... | |
void | setDestinationAddress16 (const quint32 dest) |
Sets the 16-bit address of the destination device, if known. More... | |
void | setCommandOptions (const RemoteCommandOptions options) |
Sets the remote command options. | |
quint64 | destinationAddress64 () const |
Returns the 64-bits address of the destination device. More... | |
quint16 | destinationAddress16 () const |
Returns the 16-bits address of the destination device. More... | |
RemoteCommandOptions | commandOptions () const |
Returns the RemoteATCommandRequest's options. More... | |
![]() | |
ATCommand (QObject *parent=0) | |
ATCommand's constructor. More... | |
void | setCommand (const ATCommandType command) |
Sets the AT Command. More... | |
void | setCommand (const QByteArray &command) |
Sets the AT Command from the given QByteArray. More... | |
void | setParameter (const QByteArray ¶m) |
Sets command's parameter. More... | |
ATCommandType | command () const |
Returns the AT Command. More... | |
QByteArray | parameter () const |
Returns the command's parameter. More... | |
![]() | |
XBeePacket (QObject *parent=0) | |
XBeePacket's constructor. More... | |
void | setStartDelimiter (unsigned sd) |
Sets the frame's start delimiter. By default the start delemiter is set to 0x7E. More... | |
void | setLength (unsigned length) |
Sets the frame's length. More... | |
void | setFrameType (ApiId type) |
Sets the frame's type. More... | |
void | setFrameId (quint8 id) |
Sets the frame's id. More... | |
void | setChecksum (unsigned cs) |
Sets the checksum. More... | |
bool | setPacket (const QByteArray &packet) |
Sets the packet's raw data. More... | |
QByteArray | packet () const |
Returns the frame's packet (raw data) More... | |
unsigned | startDelimiter () const |
Returns the frame's start delimiter. More... | |
quint16 | length () const |
Returns the frame-specific data length (Number of bytes between the length and the checksum) More... | |
ApiId | frameType () const |
Returns the frame's type. More... | |
quint8 | frameId () const |
Returns the frame's id. More... | |
unsigned | checksum () const |
Returns the packet's checksum. More... | |
void | escapePacket () |
bool | unescapePacket () |
Protected Attributes | |
quint64 | m_destinationAddress64 |
quint16 | m_destinationAddress16 |
RemoteCommandOptions | m_options |
Additional Inherited Members | |
![]() | |
static QString | atCommandToString (const ATCommandType command) |
Returns the given ATCommand::ATCommand into QString. More... | |
static QByteArray | atCommandToByteArray (const ATCommandType command) |
Returns the given ATCommand::ATCommand into QByteArray. More... | |
static ATCommandType | atCommandFromByteArray (const QByteArray &command) |
Returns the ATCommand::ATCommand corresponding to the given QByteArray. More... | |
![]() | |
virtual bool | parseApiSpecificData (const QByteArray &data) |
Parses the packet API specific data. More... | |
void | createChecksum (QByteArray array) |
Computes the checksum of the given QByteArray, and set it. More... | |
Detailed Description
The RemoteATCommandRequest class is used to query or set module parameters on a remote device.
For parameter changes on the remote device to take effect, changes must be applied, either by setting the apply changes options bit, or by sending an AC command to the remote.
API identifier value: API identifier value: 0x17
Member Enumeration Documentation
Constructor & Destructor Documentation
|
explicit |
RemoteATCommandRequest's constructor.
- Parameters
-
parent parent object
Member Function Documentation
|
virtual |
Assembles the packet to be able to send it.
This function is called by the XBee class, when the packet in being sent.
- Note
- Overload this function to create your own packet.
Reimplemented from QtXBee::ATCommand.
|
virtual |
Clears the packet by reseting all attributes to their default value.
- Note
- All subclass should reimplement this method.
Reimplemented from QtXBee::ATCommand.
RemoteATCommandRequest::RemoteCommandOptions QtXBee::RemoteATCommandRequest::commandOptions | ( | ) | const |
Returns the RemoteATCommandRequest's options.
- Returns
- the RemoteATCommandRequest's options
quint16 QtXBee::RemoteATCommandRequest::destinationAddress16 | ( | ) | const |
Returns the 16-bits address of the destination device.
- Returns
- the 16-bits address of the destination device
quint64 QtXBee::RemoteATCommandRequest::destinationAddress64 | ( | ) | const |
Returns the 64-bits address of the destination device.
- Returns
- the 64-bits address of the destination device
void QtXBee::RemoteATCommandRequest::setDestinationAddress16 | ( | const quint32 | dest | ) |
Sets the 16-bit address of the destination device, if known.
Set to 0xFFFE if the address is unknown, or if sending a broadcast.
- Parameters
-
dest
void QtXBee::RemoteATCommandRequest::setDestinationAddress64 | ( | const quint64 | dest | ) |
Sets the 64-bits address of the destination device.
The following addresses are also supported:
- 0x0000000000000000 - Reserved 64-bit address for the coordinator
- 0x000000000000FFFF - Broadcast address
- Parameters
-
dest
|
virtual |
Returns a debug string containing all packet's informations.
- Returns
- a debug string containing all packet's informations.
Reimplemented from QtXBee::ATCommand.
The documentation for this class was generated from the following files:
- /home/thomas/Projects/QtXBee/src/qtxb/remoteatcommandrequest.h
- /home/thomas/Projects/QtXBee/src/qtxb/remoteatcommandrequest.cpp